Tkinter is a Python-based graphical user interface library from which we can create a variety of graphical user interface applications. Using tkinter, we’ll create a text editor akin to notepad. This notepad will include a menu with options for creating new files, opening existing files, saving files, editing, and copying and pasting.
Prerequisite
- Python has been installed.
- Tkinter has been installed.
Important note: tkinter is included with Python 3.x as a standard library.
Adding menu items: Our notepad’s main menu will consist of four sections: File, Edit, Commands & Help. Our file menu item will include the following sub-menus: New, Open, Save, and Exit.
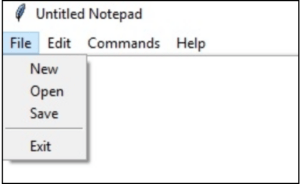
Our edit menu item will have three sub-items- cut, copy & paste
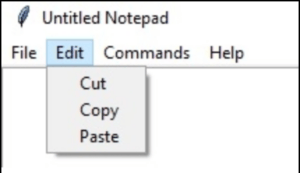
Our commands menu item will contain a single submenu item titled “About commands.”
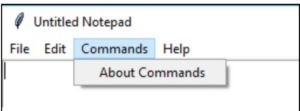
Additionally, our help menu will include a submenu titled “About notepad.”

We’re going to provide these numerous menu items and subitems by utilising the following code.
# To open new file
self.__thisFileMenu.add_command(label="New",
command=self.__newFile)
# To open a already existing file
self.__thisFileMenu.add_command(label="Open",
command=self.__openFile)
# To save current file
self.__thisFileMenu.add_command(label="Save",
command=self.__saveFile)
# To create a line in the dialog
self.__thisFileMenu.add_separator()
self.__thisFileMenu.add_command(label="Exit",
command=self.__quitApplication)
self.__thisMenuBar.add_cascade(label="File", menu=self.__thisFileMenu)
# To give a feature of cut
self.__thisEditMenu.add_command(label="Cut",
command=self.__cut)
# to give a feature of copy
self.__thisEditMenu.add_command(label="Copy",
command=self.__copy)
# To give a feature of paste
self.__thisEditMenu.add_command(label="Paste",
command=self.__paste)
# To give a feature of editing
self.__thisMenuBar.add_cascade(label="Edit", menu=self.__thisEditMenu)
# To create a feature of description of the notepad
self.__thisHelpMenu.add_command(label="About Notepad",
command=self.__showAbout)
self.__thisCommandMenu.add_command(label = "About Commands", command=self.__showCommand)
self.__thisMenuBar.add_cascade(label="Commands", menu=self.__thisCommandMenu)
self.__thisMenuBar.add_cascade(label="Help", menu=self.__thisHelpMenu)
Enhancing each Menu item’s functionality
Now that we’ve created the menu items, we’re going to add functionality to each of them. Below is a list of the features we’re going to add to this notepad (of course you can add many others too).
- Open file
- New file
- Save file
- Quit Application
- Show About
- Show Commands
- Cut
- Copy
- Paste
The following is the code for implementing the aforementioned functionality.
def __quitApplication(self):
self.__root.destroy()
# exit()
def __showAbout(self):
showinfo("About Notepad","Simple text editor like notepad using Python")
def __showCommand(self):
showinfo("Notepad", "Just Another TextPad \n Copyright \n with BSD license you can use it'")
def __openFile(self):
self.__file = askopenfilename(defaultextension=".txt", filetypes=[("All Files","*.*"),("Text Documents","*.txt")])
if self.__file == "":
# no file to open
self.__file = None
else:
# Try to open the file
# set the window title
self.__root.title(os.path.basename(self.__file) + " - Notepad")
self.__thisTextArea.delete(1.0,END)
file = open(self.__file,"r")
self.__thisTextArea.insert(1.0,file.read())
file.close()
def __newFile(self):
self.__root.title("Untitled Notepad")
self.__file = None
self.__thisTextArea.delete(1.0,END)
def __saveFile(self):
if self.__file == None:
# Save as new file
self.__file = asksaveasfilename(initialfile='Untitled.txt', defaultextension=".txt", filetypes=[("All Files","*.*"), ("Text Documents","*.txt")])
if self.__file == "":
self.__file = None
else:
# Try to save the file
file = open(self.__file,"w")
file.write(self.__thisTextArea.get(1.0,END))
file.close()
# Change the window title
self.__root.title(os.path.basename(self.__file) + " - Notepad")
else:
file = open(self.__file,"w")
file.write(self.__thisTextArea.get(1.0,END))
file.close()
def __cut(self):
self.__thisTextArea.event_generate("<>")
def __copy(self):
self.__thisTextArea.event_generate("<>")
def __paste(self):
self.__thisTextArea.event_generate("<>")
Now that we’ve added the required package, created a menu item, and added the required functionality to this notepad-like text editor that makes use of the tkinter library, we’re ready to see it in action.
The complete programme for creating a notepad text editor using tkinter is included below.
#Import os library
import os
#import everything from tkinter
from tkinter import *
#To get the space above the message
from tkinter.messagebox import *
#To get the dialog box to open when required
from tkinter.filedialog import *
class Notepad:
# Set up the root widget
__root = Tk()
__thisWidth = 500
__thisHeight = 700
__thisTextArea = Text(__root)
__thisMenuBar = Menu(__root)
__thisFileMenu = Menu(__thisMenuBar, tearoff=0)
__thisEditMenu = Menu(__thisMenuBar, tearoff=0)
__thisHelpMenu = Menu(__thisMenuBar, tearoff=0)
__thisCommandMenu = Menu(__thisMenuBar, tearoff=0)
# To add scrollbar
__thisScrollBar = Scrollbar(__thisTextArea)
__file = None
def __init__(self,**kwargs):
# icon
try:
self.__root.wm_iconbitmap("Notepad.ico")
except:
pass
# Set window size as mentioned above (the default is 300x300)
try:
self.__thisWidth = kwargs['width']
except KeyError:
pass
try:
self.__thisHeight = kwargs['height']
except KeyError:
pass
# the window text
self.__root.title("Untitled-Notepad")
# Center the window
screenWidth = self.__root.winfo_screenwidth()
screenHeight = self.__root.winfo_screenheight()
# For left-alling
left = (screenWidth / 2) - (self.__thisWidth / 2)
# For right-allign
top = (screenHeight / 2) - (self.__thisHeight /2)
# For top and bottom
self.__root.geometry('%dx%d+%d+%d' % (self.__thisWidth, self.__thisHeight, left, top))
# To make the textarea auto resizable
self.__root.grid_rowconfigure(0, weight=1)
self.__root.grid_columnconfigure(0, weight=1)
# Add controls (widget)
self.__thisTextArea.grid(sticky = N + E + S + W)
# To open new file
self.__thisFileMenu.add_command(label="New",
command=self.__newFile)
# To open a already existing file
self.__thisFileMenu.add_command(label="Open",
command=self.__openFile)
# To save current file
self.__thisFileMenu.add_command(label="Save",
command=self.__saveFile)
# To create a line in the dialog
self.__thisFileMenu.add_separator()
self.__thisFileMenu.add_command(label="Exit",
command=self.__quitApplication)
self.__thisMenuBar.add_cascade(label="File", menu=self.__thisFileMenu)
# To give a feature of cut
self.__thisEditMenu.add_command(label="Cut",
command=self.__cut)
# to give a feature of copy
self.__thisEditMenu.add_command(label="Copy",
command=self.__copy)
# To give a feature of paste
self.__thisEditMenu.add_command(label="Paste",
command=self.__paste)
# To give a feature of editing
self.__thisMenuBar.add_cascade(label="Edit", menu=self.__thisEditMenu)
# To create a feature of description of the notepad
self.__thisHelpMenu.add_command(label="About Notepad",
command=self.__showAbout)
self.__thisCommandMenu.add_command(label = "About Commands", command=self.__showCommand)
self.__thisMenuBar.add_cascade(label="Commands", menu=self.__thisCommandMenu)
self.__thisMenuBar.add_cascade(label="Help", menu=self.__thisHelpMenu)
self.__root.config(menu=self.__thisMenuBar)
self.__thisScrollBar.pack(side=RIGHT,fill=Y)
# Scrollbar will adjust automatically according to the content
self.__thisScrollBar.config(command=self.__thisTextArea.yview)
self.__thisTextArea.config(yscrollcommand=self.__thisScrollBar.set)
def __quitApplication(self):
self.__root.destroy()
# exit()
def __showAbout(self):
showinfo("About Notepad","Simple text editor like notepad using Python")
def __showCommand(self):
showinfo("Notepad", "Just Another TextPad \n Copyright \n with BSD license you can use it'")
def __openFile(self):
self.__file = askopenfilename(defaultextension=".txt", filetypes=[("All Files","*.*"),("Text Documents","*.txt")])
if self.__file == "":
# no file to open
self.__file = None
else:
# Try to open the file
# set the window title
self.__root.title(os.path.basename(self.__file) + " - Notepad")
self.__thisTextArea.delete(1.0,END)
file = open(self.__file,"r")
self.__thisTextArea.insert(1.0,file.read())
file.close()
def __newFile(self):
self.__root.title("Untitled Notepad")
self.__file = None
self.__thisTextArea.delete(1.0,END)
def __saveFile(self):
if self.__file == None:
# Save as new file
self.__file = asksaveasfilename(initialfile='Untitled.txt', defaultextension=".txt", filetypes=[("All Files","*.*"), ("Text Documents","*.txt")])
if self.__file == "":
self.__file = None
else:
# Try to save the file
file = open(self.__file,"w")
file.write(self.__thisTextArea.get(1.0,END))
file.close()
# Change the window title
self.__root.title(os.path.basename(self.__file) + " - Notepad")
else:
file = open(self.__file,"w")
file.write(self.__thisTextArea.get(1.0,END))
file.close()
def __cut(self):
self.__thisTextArea.event_generate("<>")
def __copy(self):
self.__thisTextArea.event_generate("<>")
def __paste(self):
self.__thisTextArea.event_generate("<>")
def run(self):
# Run main application
self.__root.mainloop()
# Run main application
notepad = Notepad(width=600,height=400)
notepad.run()
When we run the preceding programme, we’ll see a pop-up notepad text editor, similar to this.
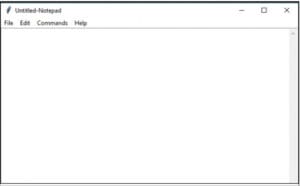
Congratulations, We created a Python Notepad, which I believe is quite cool, and I hope you enjoyed the process. As a result, if you enjoy this, please leave a comment and follow me on YouTube, Facebook, Twitter, and LinkedIn. Don’t forget to subscribe to my channel by clicking the bell icon.