A tip is money paid to the person who serves you in a restaurant as a token of appreciation for good service. A basic tip calculator is created in this project, which takes the invoice amount, type of service, and number of people as inputs. It produces a gratuity for the serving person based on the three inputs.
We’ll ask the user for help in determining the tip: We’re using a drop-down menu with quality as the options in percentage for the type of service (it’s the amount of the total bill; we’re taking this into the amount variable), and we’re using a drop-down menu with quality as the options in percentage for the type of service (it’s the amount of the total bill; we’re taking this into the amount variable) (like good, bad, excellent, etc.) Finally, we use the number of people as an input (it will help to divide the tip equally among all the person). We calculate the tip based on the user’s inputs and then print it using the console.log() function.
The total is the sum of the amount multiplied by the type of service and divided by the number of people.
Using HTML we are giving desired structure, option for the input, and submit button. With the help of CSS, we are beautifying our structure by giving colors and desired font, etc.
In the JavaScript section, we are processing the taken input and after calculating, the respective output is printed.
Filename is “index.html” :-
TIP CALCULATOR
Bill Amount
₹
How was the service ?
Total number of persons
Tip Amount
0₹
each
Filename “style.css” :-Â
body {
background-color: #006eff;
font-family: 'Raleway', sans-serif;
}
.container {
width: 350px;
height: 500px;
background-color: #fff;
position: absolute;
left: 50%;
top: 50%;
transform: translateX(-50%) translateY(-50%);
border-radius: 20px;
}
h1 {
position: absolute;
left: 50%;
top: -60px;
width: 300px;
transform: translateX(-50%);
background-color: #fffb05;
color: rgb(0, 0, 0);
font-weight: 100;
border-top-left-radius: 20px;
border-top-right-radius: 20px;
font-size: 18px;
text-align: center;
padding: 10px;
}
.wrapper {
padding: 20px;
}
input,
select {
width: 80%;
border: none;
border-bottom: 1px solid #123f66;
padding: 10px;
}
input:focus,
select:focus {
border: 1px solid #123f66;
outline: none;
}
select {
width: 88% !important;
}
button {
margin: 20px auto;
width: 150px;
height: 50px;
background-color: #f81414;
color: #fff;
font-size: 16px;
border: none;
border-radius: 5px;
}
.tip {
text-align: center;
font-size: 18px;
display: none;
}
Filename “app.js” :-
window.onload = () =>
//the function called when Calculate button is clicked.
{
/*calling a function calculateTip
which will calculate the tip for the bill.*/
document.querySelector('#calculate').onclick = calculateTip;
}
function calculateTip() {
/*assign values of ID : amount, person and service to
variables for further calculations.*/
let amount = document.querySelector('#amount').value;
let persons = document.querySelector('#persons').value;
let service = document.querySelector('#services').value;
console.log(service);
/*if statement will work when user presses
calculate without entering values. */
//so will display an alert box and return.
if (amount === '' && service === 'Select') {
alert("Please enter valid values");
return;
}
//now we are checking number of persons
if (persons === '1')
//if there is only one person then we need not to display each.
document.querySelector('#each').style.display = 'none';
else
//if there are more than one person we will display each.
document.querySelector('#each').style.display = 'block';
/*calculating the tip by multiplying total-bill and type of
service; then dividing it by number of persons.*/
//fixing the total amount upto 2 digits of decimal
let total = (amount * service) / persons;
total = total.toFixed(2);
//finally displaying the tip value
document.querySelector('.tip').style.display = 'block';
document.querySelector('#total').innerHTML = total;
}
Code combined of HTML, CSS and JavaScript in a single file:-
TIP CALCULATOR
Bill Amount
₹
How was the service ?
Total number of persons
Tip Amount
0₹
each
Output:–
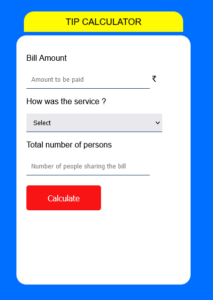

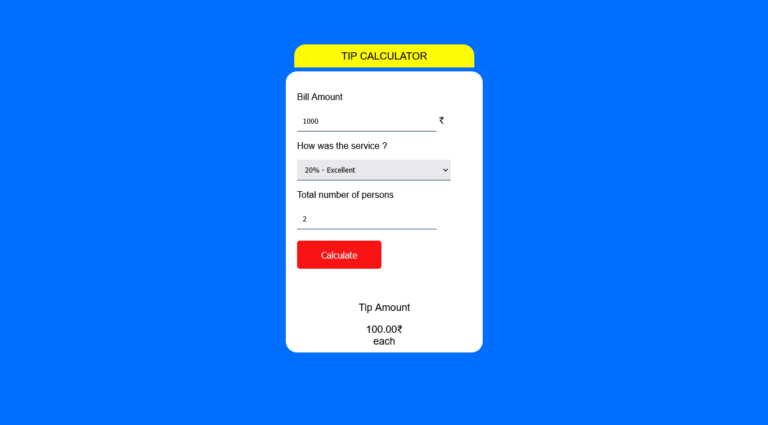
Congratulations,
We made a Tip Calculator Using HTML, CSS, and JavaScript, which I believe is rather cool, and I hope you liked the process as much as I did.
As a result, if you enjoy this, please leave a comment and follow me on YouTube, Facebook, Twitter, and LinkedIn. Don’t forget to subscribe to my channel and clicking the bell icon.